Practice
11.4 Quiz: R
What package contains the functions
select, filter & mutate
?dplyr
(ortidyverse
)
Assume I have created some R project and I read a file with the following command - what does this tell you about the folder on my computer?
- The folder containing the R project contains a subfolder called "data" and in there is a CSV file called "somefile.csv".
I want to load a csv file into R with
readRDS()
. Why isn't it working?- The read commands are specific to the file format. readRDS() is for R files, read.csv() or readr::read_csv() are needed for csv files
What do you know when you look at the command
bigfive$extraversion
?- There is a data set called
bigfive
and we're accessing a variable calledextraversion
- There is a data set called
What keywords for loops and conditionals do you remember?
- for, while, if, else, ifelse...
I want to calculate a t-test on soul-belief (yes or no) and age in our seminar data. Why won't this command work?
- When we have a grouping variable like soul_dummy, we need to use formula notation:
v02_age ~ soul_dummy
- When we have a grouping variable like soul_dummy, we need to use formula notation:
11.5 Quiz: R Markdown
- What do I need to do to make my text look like this?
- **_look like this_**
- I want to add a subtitle to my document, where might I do that?
- Add 'subtitle: "A great subtitle" ' to the YAML header
- How do I generate a section header in my document?
- With the pound sign # Header
- Oh no, my header isn't showing up right! What could have happened?
- There needs to be a space between the # and the header
11.6 Loops: Write a for-loop that outputs the first X numbers of the Fibonacci sequence ("golden ratio")
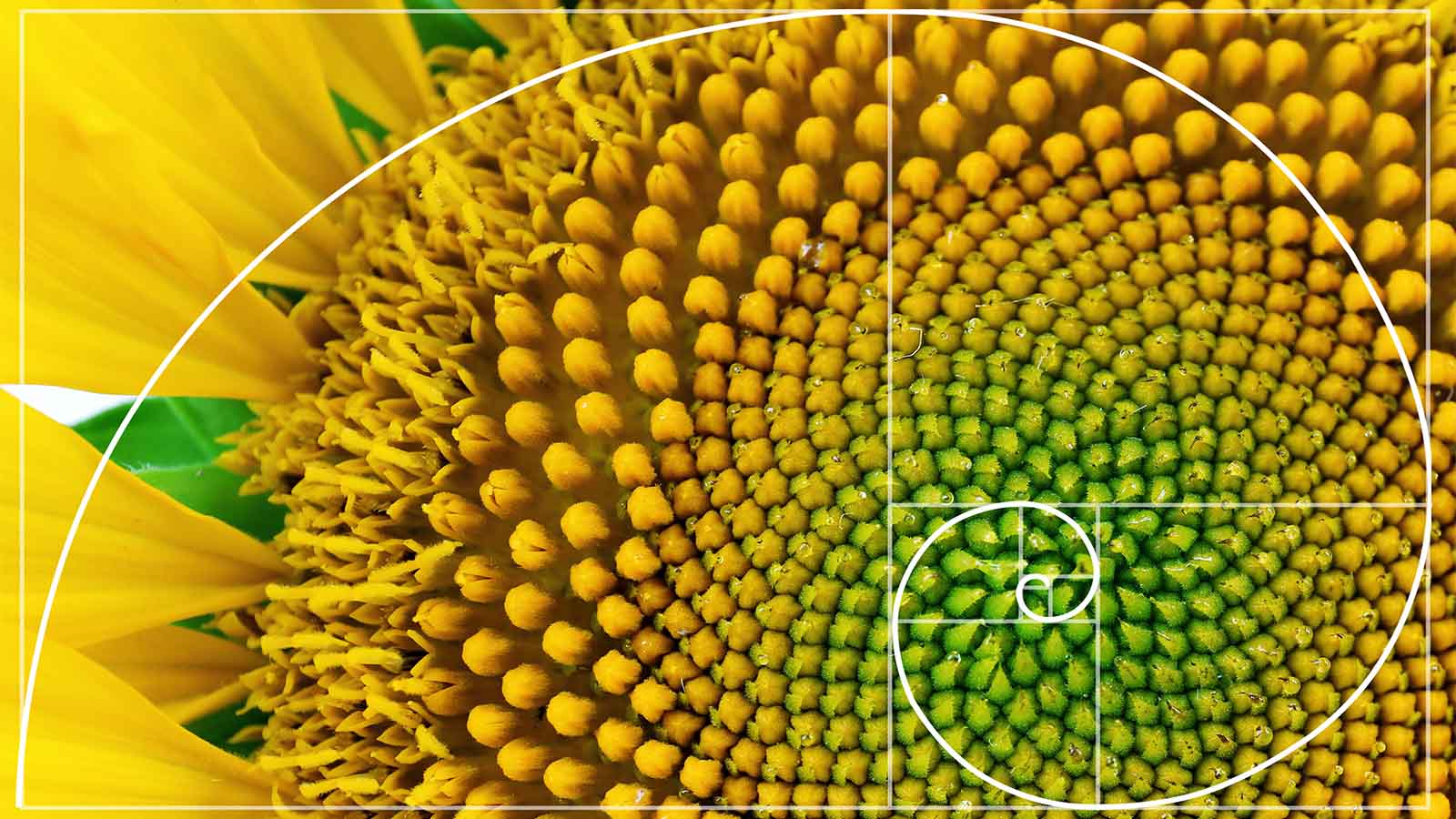
The Fibonacci sequence is defined as a sequence in which each number is the sum of the two proceeding ones. That means, starting at 1 the sequence is: 1, 1, 2, 3, 5, 8, 13... Please create it for the first 15 numbers in the Fibonacci sequence and save the numbers to a variable called fibonacci.
More info: https://en.wikipedia.org/wiki/Fibonacci_sequence.
n <- 15
# Simple and clear
fibonacci <- c(1, 1, rep(NA, n-2))
for(i in 3:n){
fibonacci[i] <- fibonacci[i-1] + fibonacci[i-2]
}
fibonacci
# [1] 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610
# Alternative with while loop
fibonacci = c(1, 1)
while(length(fibonacci) < n){
new_num <- fibonacci[length(fibonacci)] + fibonacci[length(fibonacci) - 1]
fibonacci <- c(fibonacci, new_num)
}
fibonacci
# [1] 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610
11.7 RMarkdown: Start your document in a certain way that matches the template!
Look at the example document shown below and try to re-create it! Be mindful of which elements need to be defined in the YAML header and which need to be modified in the text.
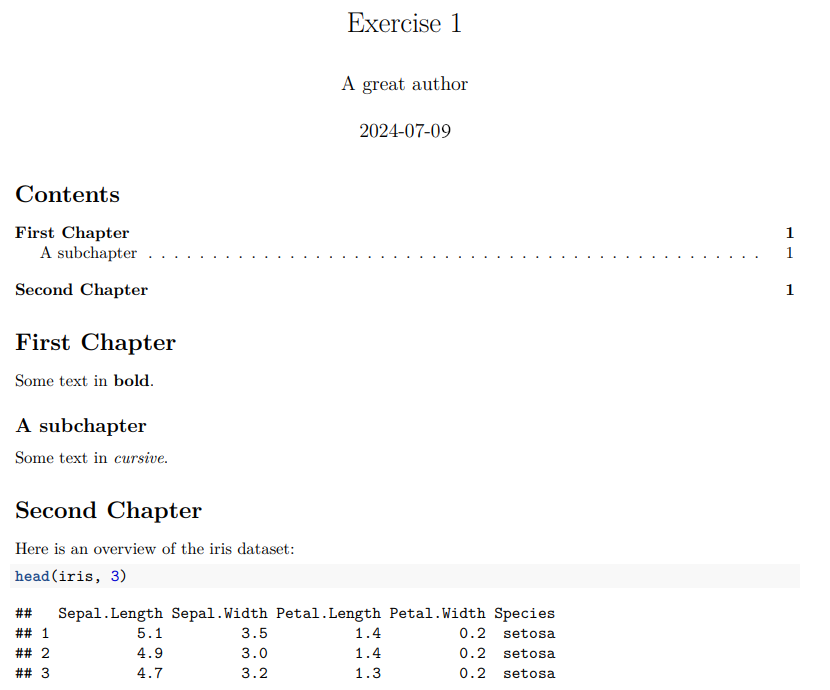
---
title: "Exercise 1"
author: "A great author"
date: "2024-07-02"
output:
pdf_document:
toc: true
---
# First Chapter
Some text in **bold**.
## A subchapter
Some text in _cursive_.
# Second Chapter
Here is an overview of the iris dataset:
``{r} # make sure to include three ` here!
head(iris, 3)
``